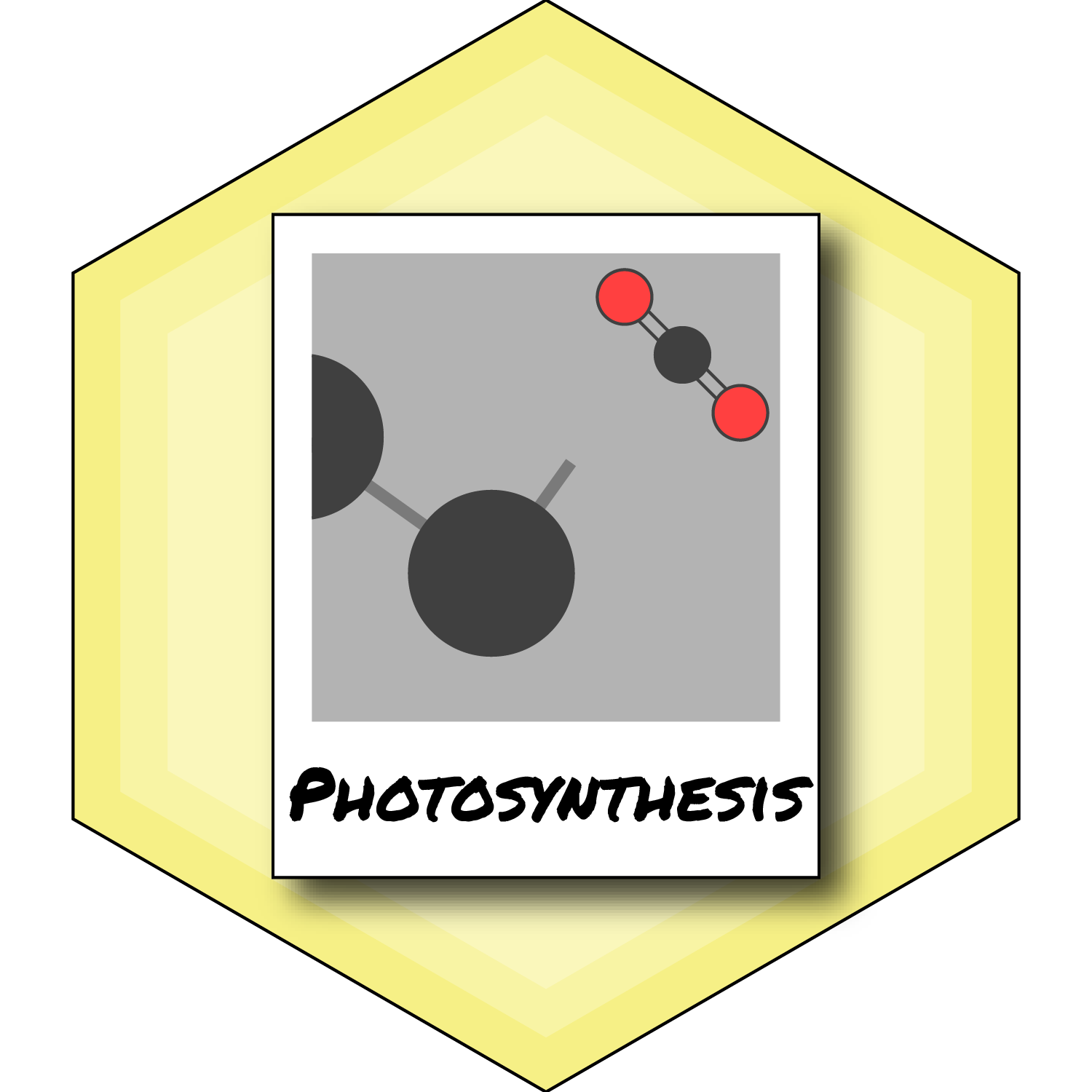
Fitting stomatal conductance models
Joseph R. Stinziano and Christopher D. Muir
2024-11-23
Source:vignettes/stomatal-conductance.Rmd
stomatal-conductance.Rmd
The package currently supports three varieties of stomatal conductance models (Ball et al. 1987; Leuning 1995; Medlyn et al. 2011).
library(dplyr)
library(photosynthesis)
round_to_nearest = function(x, values) {
sapply(x, function(y, values) {
values[which.min(abs(y - values))]
}, values = values)
}
# Read in data
dat = system.file("extdata", "A_Ci_Q_data_1.csv", package = "photosynthesis") |>
read.csv() |>
# Convert RH to a proportion
mutate(
RH = RHcham / 100,
PPFD = round_to_nearest(Qin, c(25, 50, 75, 100, 125, 375, 750, 1500))
) |>
rename(A_net = A, C_air = Ca, g_sw = gsw, VPD = VPDleaf)
# Fit the Ball-Berry stomatal conductance models
fit = fit_gs_model(
data = filter(dat, PPFD == 750),
model = c("BallBerry")
)
# Look at BallBerry model summary:
summary(fit[["BallBerry"]][["Model"]])
##
## Call:
## lm(formula = g_sw ~ gs_mod_ballberry(A_net = A_net, C_air = C_air,
## RH = RH), data = data)
##
## Residuals:
## Min 1Q Median 3Q Max
## -0.05185 -0.03045 0.01009 0.02768 0.04035
##
## Coefficients:
## Estimate Std. Error
## (Intercept) 3.659e-01 1.303e-02
## gs_mod_ballberry(A_net = A_net, C_air = C_air, RH = RH) 3.148e-06 1.423e-06
## t value Pr(>|t|)
## (Intercept) 28.076 7.63e-11 ***
## gs_mod_ballberry(A_net = A_net, C_air = C_air, RH = RH) 2.213 0.0513 .
## ---
## Signif. codes: 0 '***' 0.001 '**' 0.01 '*' 0.05 '.' 0.1 ' ' 1
##
## Residual standard error: 0.03363 on 10 degrees of freedom
## Multiple R-squared: 0.3287, Adjusted R-squared: 0.2615
## F-statistic: 4.896 on 1 and 10 DF, p-value: 0.05133
# Look at BallBerry parameters
fit[["BallBerry"]][["Parameters"]]
## g0 g1
## 1 0.3659298 3.148369e-06
# Look at BallBerry plot
# fit[["BallBerry"]][["Graph"]]
# Fit many g_sw models
fits = fit_many(dat, funct = fit_gs_model, group = "PPFD", progress = FALSE)
## Warning: `fit_many()` was deprecated in photosynthesis 2.1.3.
## This warning is displayed once every 8 hours.
## Call `lifecycle::last_lifecycle_warnings()` to see where this warning was
## generated.
## Error in nlsModel(formula, mf, start, wts) :
## singular gradient matrix at initial parameter estimates
# Look at the Medlyn_partial outputs at 750 PAR
# Model summary
summary(fits[["750"]][["Medlyn_partial"]][["Model"]])
##
## Formula: g_sw ~ gs_mod_opti(A_net = A_net, C_air = C_air, VPD = VPD, g0,
## g1)
##
## Parameters:
## Estimate Std. Error t value Pr(>|t|)
## g0 0.38778 0.03317 11.692 3.73e-07 ***
## g1 -1.09754 0.83022 -1.322 0.216
## ---
## Signif. codes: 0 '***' 0.001 '**' 0.01 '*' 0.05 '.' 0.1 ' ' 1
##
## Residual standard error: 0.04375 on 10 degrees of freedom
##
## Number of iterations to convergence: 2
## Achieved convergence tolerance: 1.49e-08
# Model parameters
fits[["750"]][["Medlyn_partial"]][["Parameters"]]
## g0 g1
## 1 0.3877773 -1.097544
# Graph
# fits[["750"]][["Medlyn_partial"]][["Graph"]]
# Compile parameter outputs for BallBerry model
# Note that it's the first element for each PAR value
# First compile list of BallBerry fits
bbmods = compile_data(data = fits, output_type = "list", list_element = 1)
# Now compile the parameters (2nd element) into a dataframe
bbpars = compile_data(data = bbmods, output_type = "dataframe", list_element = 2)
#Compile graphs
graphs = compile_data(data = bbmods, output_type = "list", list_element = 3)
# Look at 3rd graph
# graphs[[3]]
References
Ball JT, Woodrow IE, Berry JA. 1987. A model predicting stomatal conductance and its contribution to the control of photosynthesis under different environmental conditions, in Progress in Photosynthesis Research, Proceedings of the VII International Congress on Photosynthesis, vol. 4, edited by I. Biggins, pp. 221–224, Martinus Nijhoff, Dordrecht, Netherlands.
Leuning R. 1995. A critical appraisal of a coupled stomatal-photosynthesis model for C3 plants. Plant, Cell & Environment 18:339-357.
Medlyn BE, Duursma RA, Eamus D, Ellsworth DS, Prentice IC, Barton CVM, Crous KY, Angelis PD, Freeman M, Wingate L. 2011. Reconciling the optimal and empirical approaches to modeling stomatal conductance. Global Change Biology 17:2134-2144.